Image
NG-ZORRO experiments are features that are released but not yet considered stable or production ready
Developers and users can opt-in into these features before they are fully released. But breaking changes may occur with any release.
When To Use#
- When you need the browser to prioritize image loading (needs to be handled in SSR).
- When you need to optimize for HD displays (e.g. retina screens).
- When using image CDN.
- More in Image documentation
- Next steps
- Add sizes attribute and responsive support.
import { NzImageModule } from 'ng-zorro-antd/experimental/image';
Examples
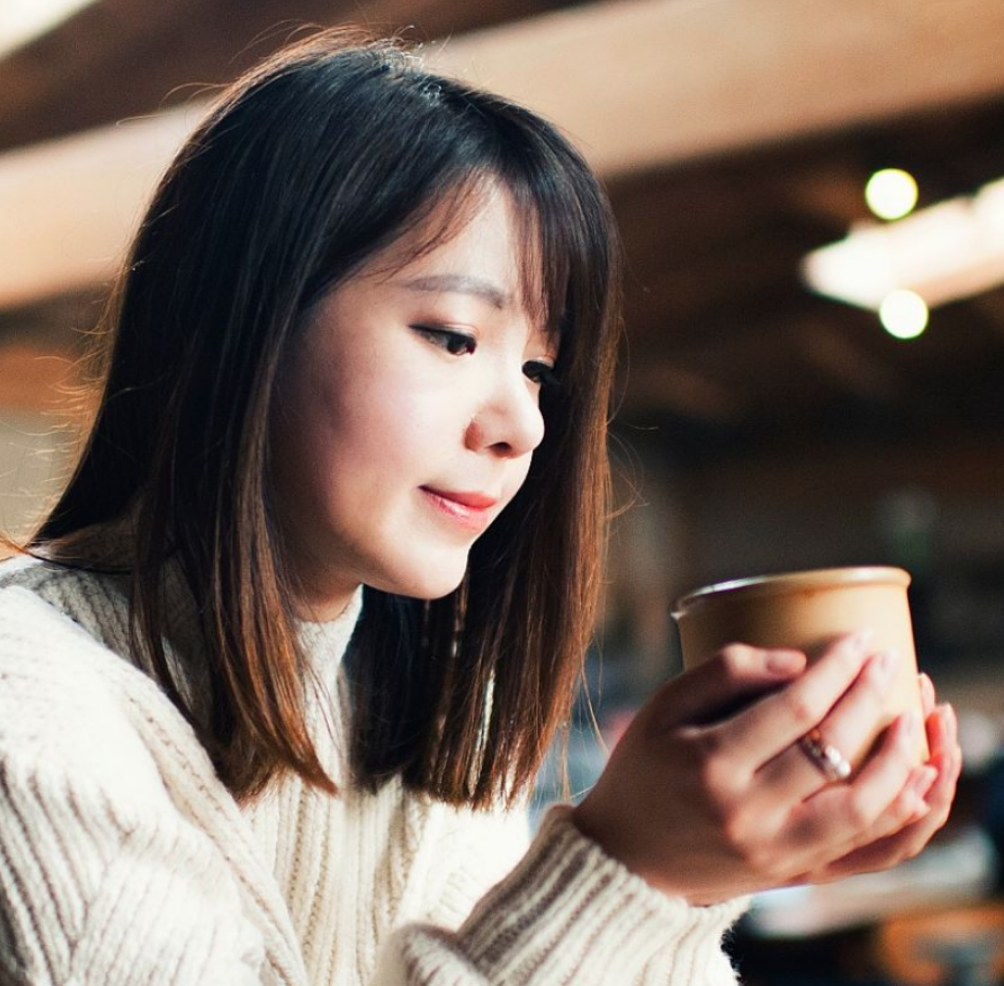
Loading...
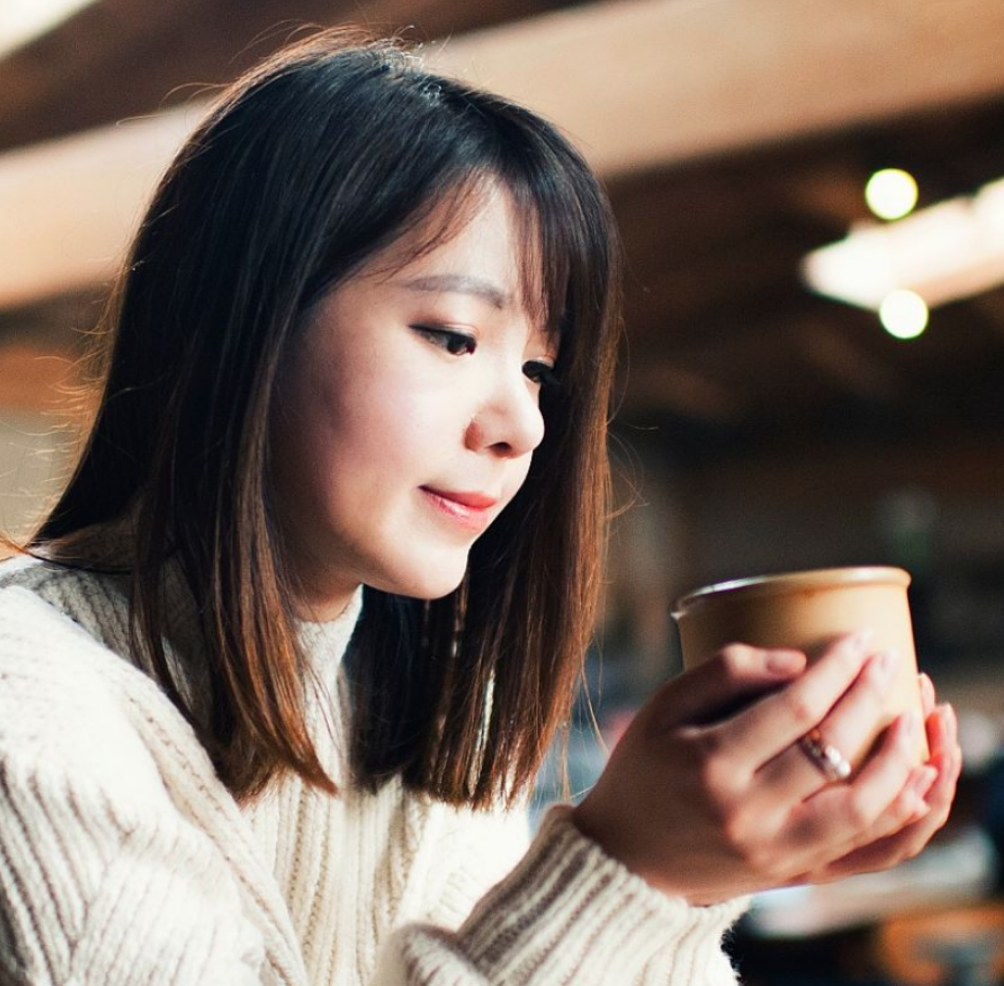
Loading...
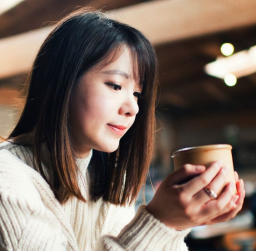
Loading...
API#
nz-image#
Property | Description | Type | Default | Global Config |
---|---|---|---|---|
nzSrc | URL | string | - | |
nzAlt | Alt | string | - | |
nzWidth | Width | number|string | auto | |
nzHeight | Height | number|string | auto | |
nzAutoSrcset | Whether to optimize image loading | boolean | false | ✅ |
nzSrcLoader | Loader | NzImageSrcLoader | defaultImageSrcLoader | ✅ |
nzPriority | Whether to add preload (only SSR) | boolean | false | ✅ |
NzImageSrcLoader#
export type NzImageSrcLoader = (params: { src: string; width: number }) => string;
Note#
nzSrcLoader#
Using nzSrcLoader
helps you to fill in key information about the requested image, such as src
and width
, which defaults to
export const defaultImageSrcLoader: NzImageSrcLoader = ({ src }) => {
return src;
};
Built-in image CND creation method
/**
* AliObjectsLoader return format
* {domain}/{src}?x-oss-process=image/resize,w_{width}
*/
export function createAliObjectsLoader(domain: string): NzImageSrcLoader;
/**
* ImgixLoader return format
* {domain}/{src}?format=auto&fit=max&w={width}
*/
export function createImgixLoader(domain: string): NzImageSrcLoader;
/**
* CloudinaryLoader return format
* {domain}/c_limit,q_auto,w_{width}/{src}
*/
export function createCloudinaryLoader(domain: string): NzImageSrcLoader;
Responsive images and preloaded images#
Using responsive images can help web pages display well on different devices. Preloading images can help you load images faster, for more information please refer to.